Overview
TNG-Hooks (/ˈting ho͝oks/) provides hooks (i.e., useState(..)
, useReducer(..)
, useEffect(..)
, etc) for decorating regular, standalone functions with useful state and effects management. Custom hooks are also supported.
TNG is inspired by the conventions and capabilities of React's Hooks, so much of TNG resembles React Hooks. The growing collections of information and examples about React's Hooks will also be useful in sparking ideas for TNG usage.
However, this is a separate project with its own motivations and specific behaviors. TNG will remain similar to React Hooks where it makes sense, but there will also be deviations as appropriate.
A new way to read docs!
Just Hover on pieces of code that are highlighted like this
and
just read about it! Instead of just looking at a bunch of boring text and scrolling
up and down :)
To read more about how this works check this repo out! or you can always check out the source of these docs!
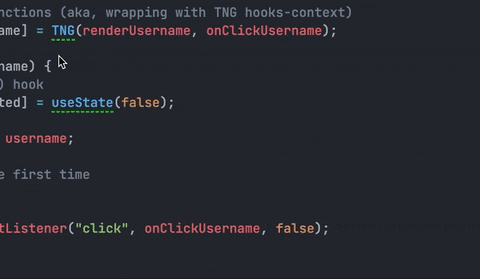
Articulated Functions
An Articulated Function is the TNG equivalent of a React function component: a regular, standalone function decorated with a TNG hooks-context, which means hooks are valid to use during its invocation.
Unlike a normal pure function, which takes all its inputs and computes output(s) without producing any side-effects, the most straightforward way to think about an Articulated Function is that it is stateful (maintains its own state) and effectful (spins off side-effects).
These will often be used to model the rendering of UI components, as is seen with React components. But Articulated Functions are useful for tracking any kind of state, as well as applying various side effects (asynchrony, Ajax calls, database queries, etc).
Similar to React's "Custom Hooks", TNG's Articulated Functions can also invoke other non-Articulated Functions, which allows those function calls to adopt the active hooks-context and use any current hooks, as if they were Articulated. These non-articulated-but-hooks-capable functions are TNG's Custom Hooks.
Quick Examples
One of the most common TNG hooks is the useState(..)
hook, which stores persistent (across invocations) state for an Articulated Function, essentially the same as React's useState(..)
hook does for a function component.
For example:
In the above snippet, activated
is persistent (across invocations) state for the renderUsername(..)
Articulated Function, and expanded
is separate persistent state for the onClickUsername(..)
Articulated Function.
activated
in the above snippet demonstrates how to perform an action just once, such as attaching a click handler to a DOM element. That works, but it's not ideal.
A much cleaner approach for handling side-effects conditionally is with the useEffect(..)
hook, which is inspired by React's useEffect(..)
hook.
For example:
In this snippet, the first useEffect( .. , [] )
passes an empty array ([]
) for its list of conditional state guards, which means that effect will only ever run the first time. The second useEffect( .., [username] )
passes [username]
for its list of conditional state guards, which ensures that its effect will only run if the username
value is different from the previous applied invocation of that effect.
TNG hooks can also be used in a non-Articulated Function, which implies it will be treated essentially like a React "Custom Hook"; to have a TNG hooks-context available, the non-Articulated Custom Hook Function must be called from an Articulated Function, or an error will be thrown.
For example:
See TNG Custom Hooks below for more information.
There are also some IMPORTANT RULES to keep in mind with using TNG hooks in your Articulated Functions and Custom Hooks.